eslint란?
lint
는 linter
라고도 하며 소스코드의 문법오류나, 버그등을 찾아주는 도구라 할 수 있습니다. 이러한 linter
는 최신 개발환경에는 필수로 자리잡았습니다. 그러므로 어떤 언어든 개발을 하기 앞서 linter
의 활용법을 잘 알아야 합니다.
자바스크립트에서 사용하는 linter
는 ESlint
입니다.
앞서 강의에서 react-native typescript 환경을 설치한 후 시작하도록 하겠습니다.
$ npx react-native init TestProject --template react-native-template-typescript
위 설정만으로도 eslint가 설치됩니다. 기본 설치 (V7.32.0) 버전이 설치되는데 최신 버전의 eslint를 설치하도고 싶다면 다음과 같은 과정을 거쳐야 합니다.
설치 방법(현재 최신 eslint 버전 v8.13.0)
$ npm install -D eslint // yarn add -D eslint
eslint를 설치했다면 환경을 설정해야합니다.
eslint의 동작에 필요한 파일
package.json
.eslintrc
또는eslintrc.js
또는eslintrc.yml
,eslintrc.json
등
package.json
가 기본으로 있어야 하며 eslint의 환경을 설정하는 .eslintrc
가 package.json
과 함께 프로젝트 루트에 있어야 합니다.
만약 eslint 환경 설정 파일이 없다면 다음 명령으로 생성하도록 합니다. 또는 기존 eslintrc 는 백업해 두고 다음 코드를 실행해도 됩니다.
$ yarn add -D @eslint/create-config
// 다음 설치는 타입스크립트를 위한 tslint 대신 eslint를 사용하기 위한 설치.
// tslint는 2019년 이후로 depreacted되어 eslint가 그 기능을 대신한다.
$ yarn add -D eslint-plugin-react@latest @typescript-eslint/eslint-plugin@latest @typescript-eslint/parser@latest
$ yarn eslint --init // 이 명령 후 질의 응답을 통해 eslint가 적용될 환경이 설정된다.
// 또한, eslint 환경 설정 파일의 형식도 정할 수 있다.
// 마지막 Would you like to install them now with npm에는 No를 선택한다.
// 또한 중간에 Browser와 node를 선택할 때는 spacebar로 체크를 헤제하거나 선택을 하여야 한다.
react-native typescript 템플릿 설치 시 eslint의 기본값
module.exports = {
root: true,
extends: '@react-native-community',
parser: '@typescript-eslint/parser',
plugins: ['@typescript-eslint'],
overrides: [
{
files: ['*.ts', '*.tsx'],
rules: {
'@typescript-eslint/no-shadow': ['error'],
'no-shadow': 'off',
'no-undef': 'off',
},
},
],
};
eslinter 설정을 json형식으로 설정했을 때
{
"env": {
"browser": true,
"es2021": true,
"node": true
},
"extends": [
"eslint:recommended",
"plugin:react/recommended",
"plugin:@typescript-eslint/recommended"
],
"parser": "@typescript-eslint/parser",
"parserOptions": {
"ecmaFeatures": {
"jsx": true
},
"ecmaVersion": 12,
"sourceType": "module"
},
"plugins": [
"react",
"@typescript-eslint"
],
"rules": {
}
}
eslinter 설정을 javascript 형식으로 했을 때 기본값
module.exports = {
"env": {
"es2021": true,
"node": true
},
"extends": [
"eslint:recommended",
"plugin:react/recommended",
"plugin:@typescript-eslint/recommended"
],
"parser": "@typescript-eslint/parser",
"parserOptions": {
"ecmaFeatures": {
"jsx": true
},
"ecmaVersion": 12,
"sourceType": "module"
},
"plugins": [
"react",
"@typescript-eslint"
],
"rules": {
}
};
eslintrc 설정
eslint의 속성들의 의미를 알아보겠습니다. 참고
- env
- 프로젝트의 실행 환경 설정입니다.
yarn eslint --init
의 질의 응답으로 설정한 환경인node
가 설정되어 있네요.browser
가 필요하면 같은 형식으로 추가해주면 됩니다. - extends - 코드 스타일 확장을 설정합니다. 예를들어 문자열에 '문자열'을 사용할 건지 "문자열"을 사용할 건지 등..
extends: '@react-native-community',
을 추가할 수 있습니다. 만약 다른 개발환경이라면 "airbnb-base" 등과 같은 다른 코딩 스타일을 찾아 설정하면 됩니다. - parser - 구문 분석기 설정을 합니다. 우리는 타입스크립트를 사용하므로
@typescript-eslint/parser
를 사용합니다. - parserOptions - 지원한 언어 옵션을 제공합니다.
jsx
는 리액트에서 사용하는 문법이므로 추가해줍니다. jsx - plugins - 처리기(processor)를 지정합니다. 우리가 작성하는 모든 코드는
javascript
파일로 변환되게 됩니다. 이를 위해 현재 어떤 코드를 작성하는지에 대한 것을 지정합니다.react
라면 react 처리가가 최종적으로 코드를 javascript 파일로 변환시킵니다.$ yarn add -D eslint-plugin-react-native
// .eslintrc { "env": { "react-native/react-native": true, }, "extends": [ "@react-native-community", "eslint:recommended", "plugin:react/recommended", "plugin:@typescript-eslint/recommended", ], ... "plugins": [ "react", "react-native" ] .... { "rules": { "react-native/no-unused-styles": 2, "react-native/split-platform-components": 2, "react-native/no-inline-styles": 2, "react-native/no-color-literals": 2, "react-native/no-raw-text": 2, "react-native/no-single-element-style-arrays": 2, } }
- 또한, react-native 관련 플러그인을 설치하여 환경을 추가하여 react-native 관련 몇가지
rules
을 추가설정할 수 있습니다.
$ npm install --save-dev eslint-plugin-react-native
- rules -
eslint
기본 제공 룰과 다양한 커스텀 룰을 설정할 수 있습니다. 룰에 부여되는 숫자는 해당 룰을 어겼을 때 어떻게 처리할 건지를 의미합니다.- 0 : 그냥둠
- 1: 경고
- 2: error
// .eslintrc
module.exports = {
"env": {
"es2021": true,
"node": true,
"react-native/react-native": true,
},
"extends": [
"eslint:recommended",
"plugin:react/recommended",
"plugin:@typescript-eslint/recommended"
],
"parser": "@typescript-eslint/parser",
"parserOptions": {
"ecmaFeatures": {
"jsx": true
},
"ecmaVersion": "latest",
"sourceType": "module"
},
"plugins": [
"react",
"react-native",
"@typescript-eslint"
],
"rules": {
"react-native/no-unused-styles": 2,
"react-native/split-platform-components": 2,
"react-native/no-inline-styles": 2,
"react-native/no-color-literals": 2,
"react-native/no-raw-text": 2,
"react-native/no-single-element-style-arrays": 2,
}
}
더 많은 설정을 문서를 보면서 천천히 하면 됩니다.
위 설정 후 react-native
의 기본 예제의 App.tsx
에
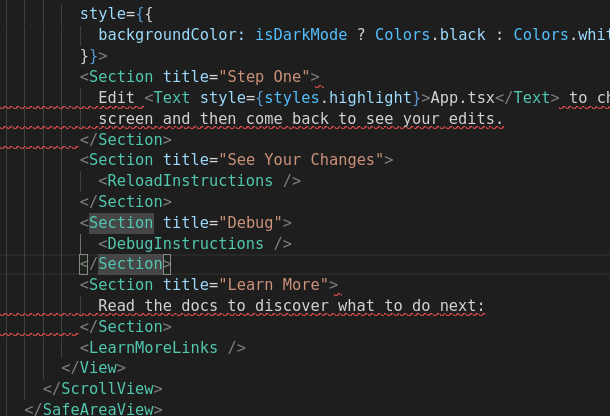
Raw text (Edit) cannot be used outside of a <Text> tageslint(react-native/no-raw-text)
가 발생합니다. 태그를 벗어나 텍스트를 작성해서 그렇습니다. 사실 이 코드는 따로 Section
이라는 컴포넌트를 만들어 결론적으로는 Text
태그로 모든 텍스트를 감싸게 되므로 문제는 없습니다. 만약 이 설정이 싫다면 이 rule
을 지우거나 해당 파일에서만 적용되지 않도록 하시면 됩니다.
eslint 룰 추가
.eslintrc.js
파일에 rules
에 다음 속성을 추가합니다.
...
"rules": {
...
curly: "error"
}
...
curly는 중괄호를 뜻한다. curly brakets 또는 curly braces라고도 합니다.
이 설정은 if문을 {}없이 쓰지 못하도록 하는 설정입니다.
const flag = true;
if (flag) console.log("true");
위와 같이 자주 사용하지만 중괄호 없이 사용할 경우 실수하기 쉽습니다.
const flag = true;
let a = 0
if (flag) a+=1;
console.log('a value changed'); // 언제나 실행됨.
flag
가 true
때만 a value changed
를 콘솔에 출력하고 싶었지만 if 문에 중괄호가 없으므로 이 출력은 if문에 포함되지 않게되어 항상 출력됩니다.